ESP32 Web Server using AJAX
ESP32 controller is programmed as a web server communicating with a client web browser using AJAX web technology, allow us to update part of web page without refreshing web page.
Why AJAX
AJAX is a developer's dream, because you can:
- Read data from a web server - after a web page has loaded
- Update a web page without reloading the page
- Send data to a web server - in the background
What is AJAX
- AJAX = Asynchronous JavaScript And XML.
- AJAX is not a programming language.
- AJAX just uses a combination of:
- XMLHttpRequest object (to exchange data asynchronously with a server)
- JavaScript and HTMLDOM (to display/interact with data)
- CSS (to style the data)
- XML (often used as the format for transferring data)
AJAX is a misleading name. AJAX applications might use XML to transport data, but it is equally common to transport data as plain text or JSON text.
AJAX allows web pages to be updated asynchronously by exchanging data with a web server behind the scenes. This means that it is possible to update parts of a web page, without reloading the whole page.
How AJAX Works
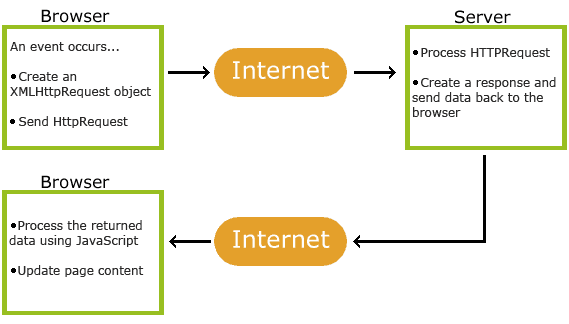
- An event occurs in a web page (the page is loaded, a button is clicked)
- An XMLHttpRequest object is created by JavaScript
- The XMLHttpRequest object sends a request to a web server
- The server processes the request
- The server sends a response back to the web page
- The response is read by JavaScript
- Proper action (like page update) is performed by JavaScript
ESP32 Web Server using AJAX
Source Code
Arduino File
//========================================================
//ESP32 Web Server: Reading Potentiometer Value using AJAX
//========================================================
#include <WiFi.h>
#include <WiFiClient.h>
#include <WebServer.h>
#include "webpage.h"
//---------------------------------------------------
WebServer server(80);
const char* ssid = "network name";
const char* password = "password";
//---------------------------------------------------
#include "handleFunctions.h"
//===================================================
void setup()
{
Serial.begin(115200);
//-------------------------------------------------
WiFi.mode(WIFI_STA);
WiFi.begin(ssid, password);
Serial.println("Connecting to WiFi");
while(WiFi.waitForConnectResult() != WL_CONNECTED)
{
delay(500); Serial.print(".");
}
Serial.print("IP address: ");
Serial.println(WiFi.localIP());
//-------------------------------------------------
server.on("/", handleRoot);
server.on("/readPOT", handlePOT);
server.begin();
Serial.println("HTTP server started");
}
//===================================================
void loop(void)
{
server.handleClient(); delay(1);
}
Header file "handleFunctions.h"
//=============================================
//Handle functions executed upon client request
//=============================================
void handleRoot()
{
server.send(200, "text/html", webpageCode);
}
//---------------------------------------
void handlePOT()
{
String POTval = String(analogRead(A0));
server.send(200, "text/plane", POTval);
}
Header file "webpage.h"
//============
//Webpage Code
//============
String webpageCode = R"***(
<!DOCTYPE html>
<head>
<title> ESP32 Web Server </title>
</head>
<html>
<!----------------------------CSS---------------------------->
<style>
body {background-color: rgba(128, 128, 128, 0.884)}
h4 {font-family: arial; text-align: center; color: white;}
.card
{
max-width: 450px;
min-height: 100px;
background: rgba(255, 0, 0, 0.521);
padding: 10px;
font-weight: bold;
font: 25px calibri;
text-align: center;
box-sizing: border-box;
color: blue;
margin:20px;
box-shadow: 0px 2px 15px 15px rgba(0,0,0,0.75);
}
</style>
<!----------------------------HTML--------------------------->
<body>
<div class="card">
<h1><span style="background-color:white">ESP32 Web Server</span></h1>
<h2>
POT Value : <span style="color:yellow" id="POTvalue">0</span>
</h2>
</div>
<h4>
<button onclick="help()">Help</button><br><br>
<div id="myDIV"> </div>
</h4>
<!-------------------------JavaScrip------------------------->
<script>
setInterval(function()
{
getPOTval();
}, 2000);
//-------------------------------------------------------
function getPOTval()
{
var POTvalRequest = new XMLHttpRequest();
POTvalRequest.onreadystatechange = function()
{
if(this.readyState == 4 && this.status == 200)
{
document.getElementById("POTvalue").innerHTML =
this.responseText;
}
};
POTvalRequest.open("GET", "readPOT", true);
POTvalRequest.send();
}
//-------------------------------------------------------
function help()
{
var x = document.getElementById("myDIV");
var message = "POT connected to ADC0 : 12-bit value (0 ---> 4095)";
if (x.innerHTML == "") x.innerHTML = message;
else x.innerHTML = "";
}
</script>
</body>
</html>
)***";